-
属性常量
使对象中的属性不可修改,删除
const obj = { name: 'wahaha' } Object.defineProperty(obj, 'name', { writable: false, // 禁止修改属性值 configurable: false, // 禁止配置 }) obj.name = 'cinob' console.log(obj.name) // wahaha delete obj.name // false console.log(obj.name) // wahaha
-
不可扩展
让一个对象变的不可扩展,也就是永远不能再添加新的属性
const obj = { name: 'wahaha' } Object.preventExtensions(obj) obj.age = 18 obj.age // undefined
-
封闭对象
阻止添加新属性并将所有现有属性标记为不可配置, 相当于
Object.preventExtensions
+ 所有属性configurable:false
const obj = { name: 'wahaha' } Object.seal(obj) obj.age = 18 obj.age // undefined delete obj.name // false console.log(obj.name) // wahaha
-
冻结对象
不能向这个对象添加新的属性,不能删除已有属性,不能修改该对象已有属性的可枚举性、可配置性、可写性,以及不能修改已有属性的值,相当于
Object.seal
+ 所有属性writable:false
const obj = { name: 'wahaha' } Object.seal(obj) obj.age = 18 obj.age // undefined obj.name = 'cinob' console.log(obj.name) // wahaha delete obj.name // false console.log(obj.name) // wahaha
js中不可变对象的实现方法
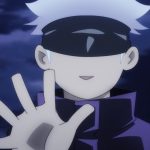
0
赏
请作者吃个鸡腿!
扫一扫支付
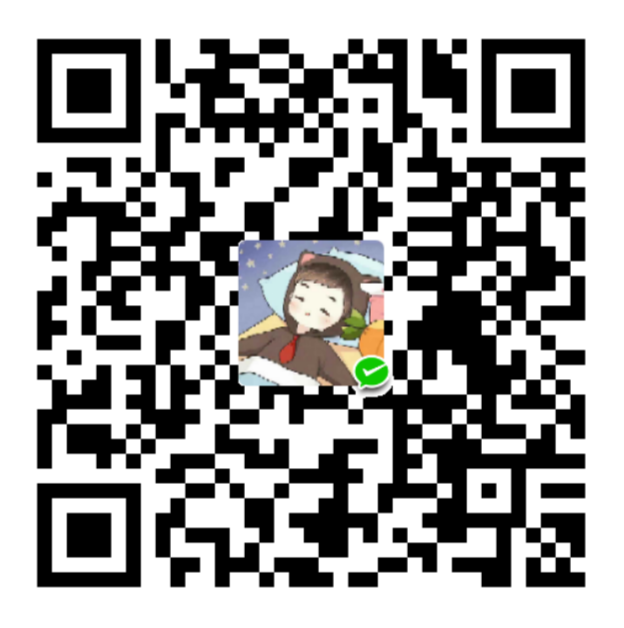
评论
还没有任何评论,你来说两句吧!